Issue #46
Hi there,
welcome to the 46th issue of the newsletter! Today among other things I'm summarizing the updates in Xcode and Swift that affect code and project quality. Hope you enjoy the reading 😊
Iterating over some elements
Here's how to iterate over only some elements in the array. Both options, for-case-let and for-in-if, are more efficient than filtering the array and iterating on the filtered results 🙌
Swift has a `for-case-let` syntax, which is equivalent to the `for-in...if-let` syntax. The former makes code cleaner but less expressive.
— Lee Kah Seng (@Lee_Kah_Seng) March 29, 2023
Personally, I would prefer to use the latter syntax to keep my code expressive. I wonder how commonly the `for-case-let` syntax is used. 🤔 pic.twitter.com/Fngk9h4e40
Xcode 14.3 & Swift 5.8
There's a bunch of improvements available in the new Swift and Xcode. Here are a few things that caught my attention:
Implicit self
- Implicit
self
is now permitted forweak self
captures, afterself
is unwrapped:
class ViewController {
let button: Button
func setup() {
button.tapHandler = { [weak self] in
guard let self else { return }
dismiss() // refers to `self.dismiss()`
}
}
func dismiss() { ... }
}
Debugging previews with print
print
output now appears in the console for SwiftUI Previews by selecting “Preview” tab in the console.
Previews in modular dependencies
- Fixed: Previews can fail when previewing a file inside of a Swift package target that is the dependency of an executable target.
Waiting for expectations
- The
timeout
argument ofXCTestCase.wait(for:timeout:enforceOrder:)
and related methods is now optional—if you don’t specify it, the function waits indefinitely (until the overall test times out.) To ensure reasonable execution time, set an appropriate value for theexecutionTimeAllowance
property of the runningXCTestCase
instance (self
). XCTestCase.wait(for:timeout:enforceOrder:)
and related methods are now marked unavailable in concurrent Swift functions because they can cause a test to deadlock. Instead, you can use the new concurrency-safeXCTestCase.fulfillment(of:timeout:enforceOrder:)
method.
Test plans
- Xcode now defaults to using a test plan for new projects. Learn more about test plans in the documentation: Improving code assessment by organizing tests into test plans
Read about everything new here (this links directly to the section about Swift but you can scroll up and down to read about all the changes in Xcode):
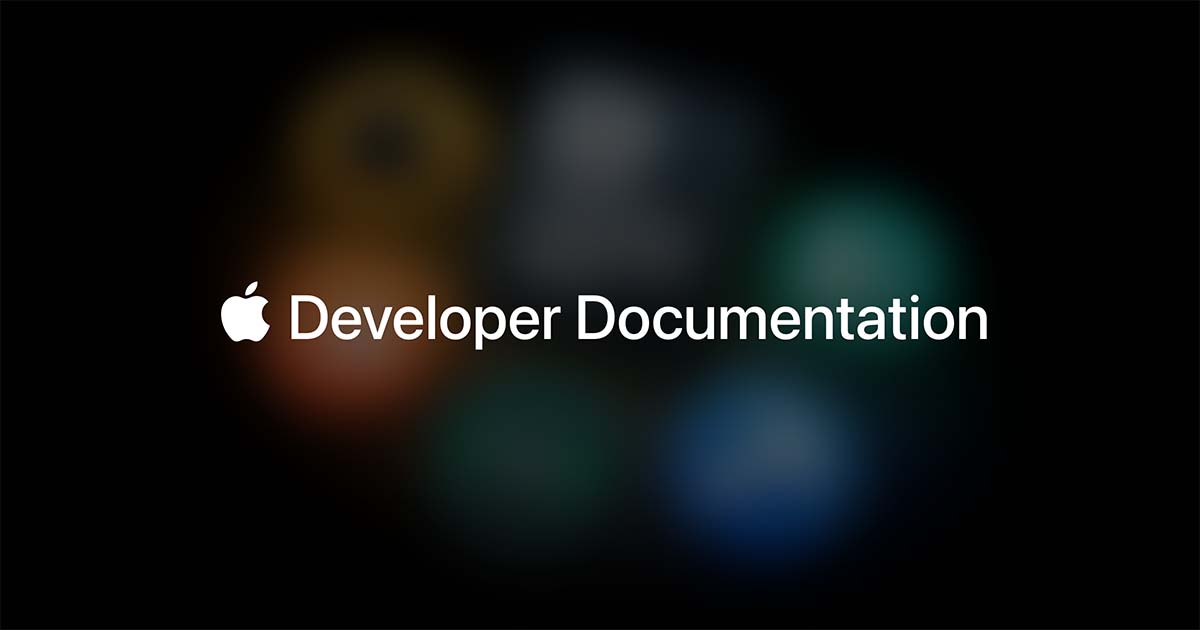
✌️
Alright, that's it for today.
I'm curious if you found one of the tips particularly interesting - let me know by replying to this email!