Issue #44
Hi there,
Welcome to the 44th issue of iOS Code Review! Today I'm sharing five topics that caught my attention during the past couple of weeks. Enjoy the reading!
In-app subscriptions are a pain to implement, hard to test, and full of edge cases. RevenueCat makes it simple by providing a backend and a wrapper around Apple's StoreKit and Google Play Billing. Get started for free.
Learn more
Structuring unit tests
To prevent our complex unit tests from turning into a mush of hard-to-read code, we can annotate the tests using the Given-When-Then technique:
// Given
let mockStorage = Storage(value: "123")
let sut = Cache(storage: mockStorage)
// When
sut.clear()
// Then
XCTAssertEqual(mockStorage.value, nil)
This is a tiny example, but a real saver for more complex test scenarios.
Read more by Martin Fowler:
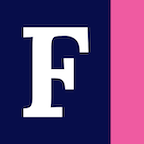
Don't change default params when subclassing
This unexpected behavior came as a total surprise for me - when we override a method with default parameters, sometimes the default parameters of the superclass's method will be used. So we should keep the default values the same in the override's method signature to avoid surprises and bugs.
Delegate naming conventions
The delegate pattern is familiar to iOS developers, but naming of the delegate methods can differ. A good practice is to include the sender parameter, and make it clear in the function name that it's a delegate method, so it stands out at the implementer side.
Read about good naming conventions for delegate methods by John Sundell:
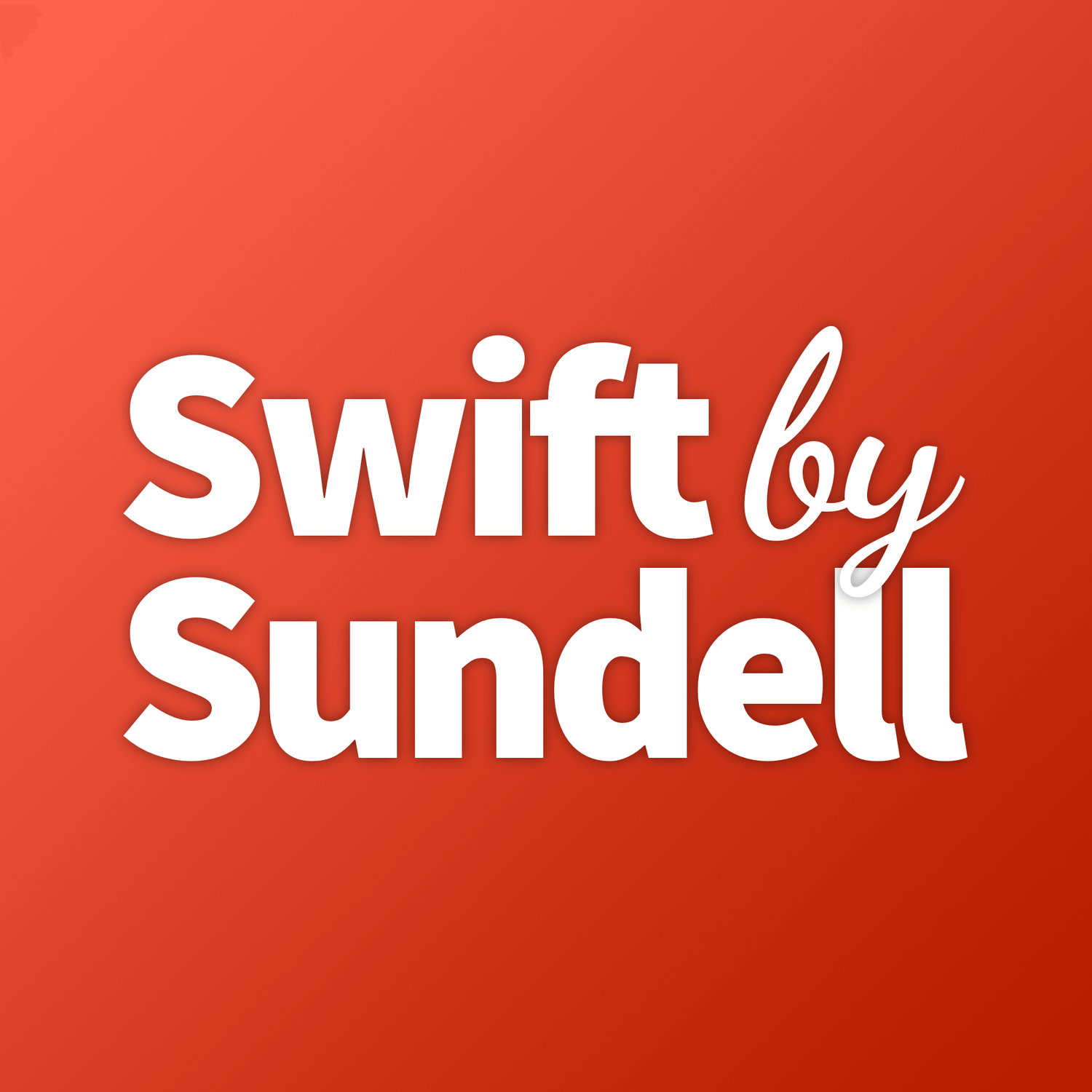
Fixing file header template for packages
Xcode still doesn't add proper filename, project name and copyright into new files created in SPM packages. Here's a short article explaining how to finally fix that:
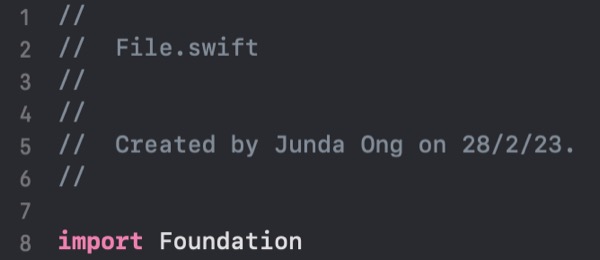
Learn about SwiftUI view lifecycle
The demo project you didn't know you needed :)
✌️
Alright, that's it for today! Thank you to RevenueCat for sponsoring this issue ❤️
I'm curious if you found one of the tips particularly interesting - let me know by replying to this email!